Docker Containerization: A Complete Guide
Master Docker containerization with this comprehensive guide covering everything from basic concepts to advanced deployment strategies.
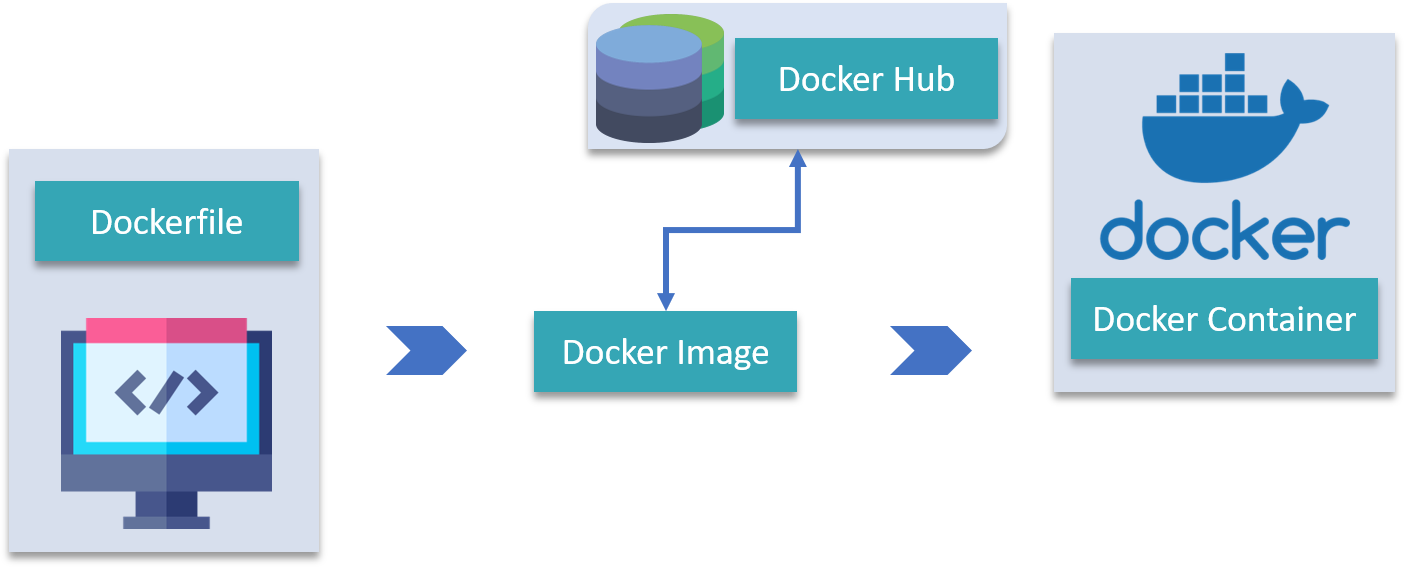
Docker Containerization: A Complete Guide
Docker has revolutionized how we build, package, and deploy applications. In this comprehensive guide, we’ll explore everything you need to know about containerization with Docker.
What is Docker?
Docker is a platform for developing, shipping, and running applications in containers. Containers allow you to package your application and its dependencies into a standardized unit for software development.
Basic Docker Commands
Here are some essential Docker commands you’ll use daily:
# Build an image
docker build -t myapp:latest .
# Run a container
docker run -d -p 8080:80 myapp:latest
# List running containers
docker ps
# Stop a container
docker stop container_id
Dockerfile Best Practices
Here’s an example of a well-structured Dockerfile:
# Use multi-stage builds
FROM node:18-alpine AS builder
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
RUN npm run build
# Production stage
FROM node:18-alpine
WORKDIR /app
COPY --from=builder /app/dist ./dist
COPY package*.json ./
RUN npm install --production
EXPOSE 3000
CMD ["npm", "start"]
Docker Compose
Docker Compose helps you define and run multi-container applications:
version: "3.8"
services:
web:
build: .
ports:
- "3000:3000"
environment:
- NODE_ENV=production
db:
image: postgres:14
environment:
- POSTGRES_PASSWORD=secret
volumes:
- postgres_data:/var/lib/postgresql/data
volumes:
postgres_data:
Container Orchestration
For production deployments, consider using container orchestration tools:
- Kubernetes: The most popular container orchestrator
- Docker Swarm: Built into Docker
- Amazon ECS: Managed container service
Best Practices
-
Use Multi-stage Builds
- Reduce final image size
- Separate build and runtime environments
-
Optimize Layer Caching
- Order Dockerfile instructions carefully
- Use .dockerignore effectively
-
Security Considerations
- Use official base images
- Scan for vulnerabilities
- Run containers as non-root
Monitoring and Logging
# View container logs
docker logs container_id
# Monitor container resources
docker stats container_id
Conclusion
Docker has become an essential tool in modern software development. By following these best practices and understanding the core concepts, you’ll be well-equipped to containerize your applications effectively.
Remember to:
- Keep images small and secure
- Use multi-stage builds
- Implement proper logging
- Follow security best practices
- Consider orchestration for production
Stay tuned for more Docker tips and tricks!