Infrastructure as Code with Terraform: A Complete Guide
Learn how to manage your cloud infrastructure using Terraform, from basic concepts to advanced state management and module development.
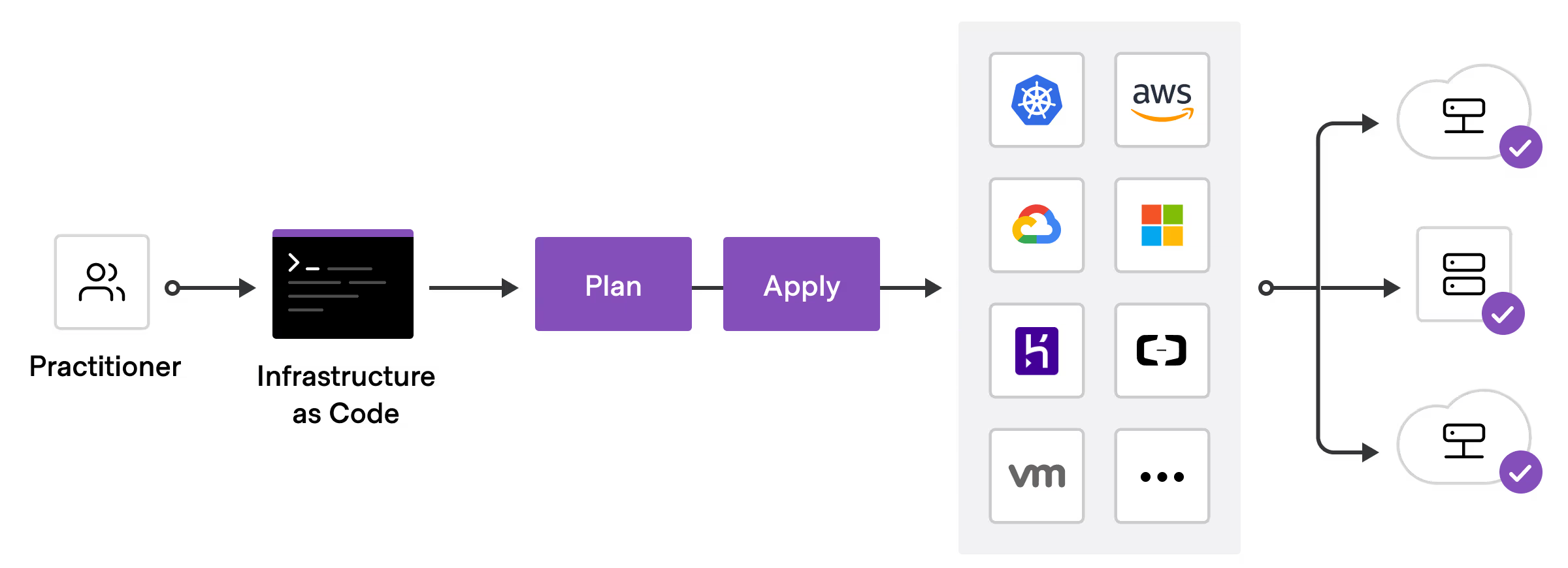
Infrastructure as Code with Terraform: A Modern Approach
Infrastructure as Code (IaC) has transformed how we manage and provision cloud resources. Terraform, by HashiCorp, has emerged as a leading tool in this space. Let’s explore how to effectively use Terraform for your infrastructure needs.
Understanding Infrastructure as Code
IaC allows you to manage your infrastructure using code, providing consistency, version control, and automation capabilities.
Terraform Basics
1. Core Concepts
- Providers
- Resources
- Data Sources
- Variables and Outputs
- State Management
2. HCL Syntax
# Provider configuration
provider "aws" {
region = "us-west-2"
}
# Resource definition
resource "aws_instance" "web" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "web-server"
Environment = "production"
}
}
Best Practices
1. Project Structure
project/
├── main.tf
├── variables.tf
├── outputs.tf
├── terraform.tfvars
└── modules/
└── vpc/
├── main.tf
├── variables.tf
└── outputs.tf
2. State Management
- Use remote state storage
- Implement state locking
- Separate state per environment
3. Module Design
- Create reusable modules
- Use consistent naming
- Document inputs and outputs
Real-World Example
VPC with Multiple Subnets
module "vpc" {
source = "./modules/vpc"
name = "production-vpc"
cidr = "10.0.0.0/16"
azs = ["us-west-2a", "us-west-2b"]
private_subnets = ["10.0.1.0/24", "10.0.2.0/24"]
public_subnets = ["10.0.101.0/24", "10.0.102.0/24"]
enable_nat_gateway = true
single_nat_gateway = false
tags = {
Environment = "production"
Terraform = "true"
}
}
Security Considerations
-
Access Management
- Use IAM roles
- Implement least privilege
- Secure state files
-
Sensitive Data
- Use encrypted variables
- Utilize AWS KMS
- Implement secure backends
CI/CD Integration
-
Pipeline Setup
- Automated planning
- Review processes
- Controlled applies
-
Testing
- Validate configurations
- Test modules
- Check compliance
Common Patterns
1. Workspaces
- Development
- Staging
- Production
2. Resource Naming
locals {
name_prefix = "${var.project}-${var.environment}"
}
resource "aws_s3_bucket" "data" {
bucket = "${local.name_prefix}-data"
# ... other configurations
}
Troubleshooting Tips
-
State Issues
- State locking problems
- State corruption
- Import existing resources
-
Plan/Apply Failures
- Dependency issues
- Provider errors
- Resource conflicts
Conclusion
Terraform provides a powerful way to manage infrastructure as code. By following these best practices and patterns, you can build maintainable and scalable infrastructure configurations.
Remember to:
- Version control your code
- Use modules for reusability
- Implement proper state management
- Follow security best practices
- Automate your workflows